Unexpected Reserved Word ‘Await’ Error In JavaScript
Unexpected Reserved Word 'Await' Error In JavaScript
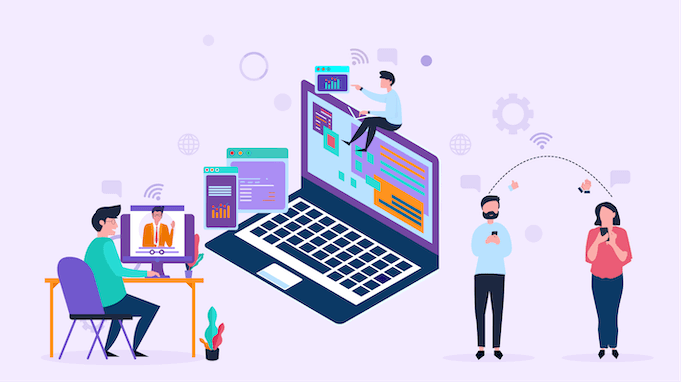
JavaScript is a popular programming language often used on the web. One of the challenges that programmers face when using JavaScript is dealing with errors. In this blog article, we’ll break down the steps to take in order to combat errors that are thrown while using the language.
Have you ever found that there is an “Await” error in JavaScript? It is normal for you to find a message of “Uncaught SyntaxError: await is not a known keyword” in the Chrome console. The cause of this error is that JavaScript didn’t support the new features of ES2017/ES8.
What is an await?
An await is a keyword in JavaScript that allows for asynchronous programming. When used within a function, an await will pause the execution of the function until the awaited asynchronous operation has been completed. This enables developers to write code that is more readable and easier to follow.
One common use for the await keyword is with promises. A promise represents an asynchronous operation that will eventually resolve to a value. By using the await keyword, you can write code that looks like it is synchronous even though it is actually asynchronous.
For example, imagine you have a list of users that you need to fetch from an API. Without using async/await, your code might look something like this:
const fetch Users = () => {
const response = fetch(‘https://jsonplaceholder.typicode.com/users’);
const data = response.json();
return data;
}
const users = fetchUsers();
console.log(users); // [{…}, {…}, {…}, {…}, {…}]
In the example above, the code fetches a list of users from an API and logs the result to the console. Even though the fetchUsers() function is asynchronous, it appears to be synchronous because it does not use any async/await keywords.
However, if we were to use async/await within our fetchUsers() function, our code would look like this:
If you receive an “Unexpected reserved word ‘await'” error in JavaScript, it is because the keyword await is a reserved word in JavaScript and not yet available for use. The await keyword is only available in JavaScript since version 1.8.5.
In order to use the await keyword, you must first declare it with the var keyword:
var await = require(“await”);
You can then use the await keyword within a function:
async function myFunc() {
// … your code here …
}
What does ‘Unexpected reserved word ‘await’ error in JavaScript.
If you’re getting the “Unexpected reserved word ‘await'” error in JavaScript, it’s likely because you’re using an async function without the await keyword. The await keyword can only be used inside an async function, and it tells the JavaScript engine to wait for the async function to complete before moving on to the next line of code.
If you’re not using an async function, you can fix this error by simply removing the await keyword from your code. However, if you are using an async function, you’ll need to add the await keyword before any asynchronous code inside that function.
If you’re getting an “unexpected reserved word ‘await'” error in JavaScript, it’s likely because you’re using an async function without the await keyword. The await keyword can only be used inside of an async function, and it tells the JavaScript engine to wait for the async function to complete before moving on to the next line of code.
If you’re not using an async function, you’ll need to remove the await keyword from your code. If you are using an async function, make sure you’re using the await keyword inside of it.
How to fix the Unexpected Reserved Word Await Error in JavaScript?
If you receive the “unexpected reserved word ‘await'” error when using the await keyword in JavaScript, there are a few potential causes:
1. You’re using an async function without the await keyword
2. You’re using an arrow function with the async keyword
3. You’re using the await keyword outside of an async function
4. Your code is not transpiling correctly
To fix this error, you’ll need to ensure that you’re using the await keyword correctly. If you’re using an async function, make sure to use the await keyword before any asynchronous calls. If you’re using an arrow function with the async keyword, make sure to put the parenthesis after the async keyword (e.g. const foo = async () => {}). Lastly, if you’re using the await keyword outside of an async function, make sure to wrap your code in an async function (e.g. const foo = async () => { await bar(); }).
If you’re getting the “unexpected reserved word ‘await'” error in JavaScript, it means that you’re using the await keyword outside of an async function. The await keyword can only be used inside an async function, and only after the async keyword has been used to declare the function. Here’s an example of how to use the await keyword correctly:
async function myAsyncFunction() {
// Some code goes here…
const result = await somePromise();
// More code goes here…
}
Conclusion
In conclusion, the ‘await’ keyword is a reserved word in JavaScript and should not be used as an identifier. If you encounter this error, there are a few ways to fix it, which include renaming the variable or function containing the ‘await’ keyword, or adding quotes around it.
In this article, we looked at the reserved word await error in JavaScript and how to fix it. We saw that this error can be caused by a number of things, but the most common cause is simply forgetting to add the async keyword before using the await keyword. With that said, there are other potential causes of this error, so be sure to check your code carefully if you run into this issue. Thanks for reading!